Собственно здравствуйте дорогие форумчане, а также гости.
Разберем мы такую тему как мини форум.
Сразу прилагаю скриншот:
Разберем мы такую тему как мини форум.
Сразу прилагаю скриншот:
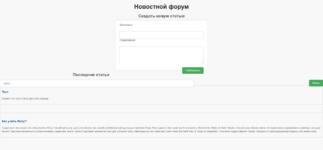
Начнем с идеи. Мне давно хотелось что то на форум похожее создать. И я, отсталый человек, только вчера узнал об ChatGPT. Дальше я думаю вы поняли
Далее, я начал экспериментировать с запросами и т. д.
И первое что я вам покажу это HTML код. Он достаточно прост, семантики нет, содержит: заголовки, форму для создания тем, форму для поиска по темам и наконец js код который показывать все темы и делает живой поиск (функцию)
HTML:
<!DOCTYPE html>
<html lang="ru">
<head>
<meta charset="UTF-8">
<title>Новостной форум</title>
<style>
/* Add your CSS styles here */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f8f8f8; /* Light grey background color */
color: #555; /* Dark grey text color */
}
h1, h2 {
text-align: center;
}
h1 {
font-size: 36px;
margin: 20px 0;
color: #222; /* Darker grey text color for headings */
}
h2 {
font-size: 24px;
margin: 10px 0;
}
form {
max-width: 500px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff; /* White background color for the form */
}
label {
display: block;
margin-bottom: 10px;
font-size: 16px;
}
input[type="text"], textarea {
width: 100%;
padding: 12px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
font-size: 16px;
resize: vertical;
}
input[type="submit"] {
background-color: #4caf50; /* Green background color for the submit button */
color: white;
padding: 12px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
float: right;
}
input[type="submit"]:hover {
background-color: #45a049; /* Darker green color for the submit button on hover */
}
.article {
border: 1px solid #ccc;
margin: 10px 0;
padding: 10px;
}
.article-title {
font-size: 18px;
font-weight: bold;
color: #006699; /* Blue color for article titles */
}
.article-content {
font-size: 14px;
line-height: 1.5;
}
input[type="text"]#search {
width: 60%;
padding: 12px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box;
font-size: 16px;
float: left;
}
button#search-button {
background-color: #4caf50; /* Green background color for the search button */
color: white;
padding: 12px 20px;
border: none;
border-radius: 4px;
cursor: pointer;
float: right;
}
button#search-button:hover {
background-color: #45a049; /* Darker green color for the search button on hover */
}
</style>
</head>
<body>
<h1>Новостной форум</h1>
<h2>Создать новую статью</h2>
<form action="/create-article.php" method="post">
<label for="title">Заголовок:</label><br>
<input type="text" id="title" name="title" required><br>
<label for="content">Содержание:</label><br>
<textarea id="content" name="content" rows="4" cols="50" required></textarea><br><br>
<input type="submit" value="Опубликовать">
</form>
<h2>Последние статьи</h2>
<p><input type="text" id="search" placeholder="Поиск...">
<button id="search-button">Искать</button><br>
<div id="articles">
<!-- Articles will be added here -->
</div>
<script>
// Fetch the list of articles from the web server and display them on the page
fetch('/articles.php')
.then(response => response.json())
.then(articles => {
const articlesDiv = document.getElementById('articles');
articles.forEach(article => {
const div = document.createElement('div');
div.classList.add('article');
div.innerHTML = `
<h3 class="article-title">${article.title}</h3>
<p class="article-content">${article.content}</p>
`;
articlesDiv.appendChild(div);
});
});
</script>
<script>
window.onload = function() {
// Get the search input and button
var searchInput = document.getElementById('search');
var searchButton = document.getElementById('search-button');
// Add an event listener to the search button
searchButton.addEventListener('click', function() {
// Get the search query
var query = searchInput.value.toLowerCase();
// Get the articles
var articles = document.getElementsByClassName('article');
// Loop through the articles and hide those that don't match the search query
for (var i = 0; i < articles.length; i++) {
var title = articles[i].getElementsByClassName('article-title')[0].textContent.toLowerCase();
var content = articles[i].getElementsByClassName('article-content')[0].textContent.toLowerCase();
if (title.indexOf(query) === -1 && content.indexOf(query) === -1) {
articles[i].style.display = 'none';
} else {
articles[i].style.display = 'block';
}
}
});
};
</script>
</body>
</html>
Кстати, там еще стили есть наверху
Так, дальше перейдем к PHP коду обработки тем и добавление в базу данных
Он принимает POST-запрос, проверяет его на плохие слова и выводит ответ: либо опубликована либо нет
PHP:
<?php
// Connect to the database
$host = "localhost";
$username = "user";
$password = "pass";
$dbname = "name";
$conn = mysqli_connect($host, $username, $password, $dbname);
if (!$conn) {
die("Коннекция упала: " . mysqli_connect_error());
}
// Load the list of bad words
$bad_words_en = file('bad_words_en.txt', FILE_IGNORE_NEW_LINES | FILE_SKIP_EMPTY_LINES);
$bad_words_ru = file('bad_words_ru.txt', FILE_IGNORE_NEW_LINES | FILE_SKIP_EMPTY_LINES);
// Handle the POST request
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$title = mysqli_real_escape_string($conn, $_POST['title']);
$content = mysqli_real_escape_string($conn, $_POST['content']);
// Check for bad words in the title and content
$title_is_clean = true;
foreach ($bad_words_en as $word) {
if (stripos($title, $word) !== false) {
$title_is_clean = false;
break;
}
}
foreach ($bad_words_ru as $word) {
if (stripos($title, $word) !== false) {
$title_is_clean = false;
break;
}
}
$content_is_clean = true;
foreach ($bad_words_en as $word) {
if (stripos($content, $word) !== false) {
$content_is_clean = false;
break;
}
}
foreach ($bad_words_ru as $word) {
if (stripos($content, $word) !== false) {
$content_is_clean = false;
break;
}
}
if ($title_is_clean && $content_is_clean) {
// Insert the new article into the database
$sql = "INSERT INTO articles (title, content) VALUES ('$title', '$content')";
if (mysqli_query($conn, $sql)) {
echo "Статья успешно создана";
} else {
echo "Error: " . $sql . "<br>" . mysqli_error($conn);
}
} else {
echo "Error: Ваша статья содержит плохие слова. Она не может быть опубликована";
}
}
// Close the connection
mysqli_close($conn);
?>
С принятием тем мы закончили. Но как же высвечиваем статьи? Все легче чем вам кажется

Смотрите) Просто принимаем гет-запрос что нужно дать все статьи а дальше переформатируем базу в список и отправляем обратно
PHP:
<?php
// Connect to the database
$host = "localhost";
$username = "user";
$password = "pass";
$dbname = "name";
$conn = mysqli_connect($host, $username, $password, $dbname);
if (!$conn) {
die("Коннекция упала: " . mysqli_connect_error());
}
// Query the database for the list of articles
$result = mysqli_query($conn, "SELECT * FROM articles");
$articles = [];
while($row = mysqli_fetch_assoc($result)) {
$articles[] = $row;
}
// Return the articles in JSON format
header('Content-Type: application/json');
echo json_encode($articles);
// Close the connection
mysqli_close($conn);
?>
Вот собственно и на сегодня все. Надеюсь вам понравилась моя статья, до новых встреч